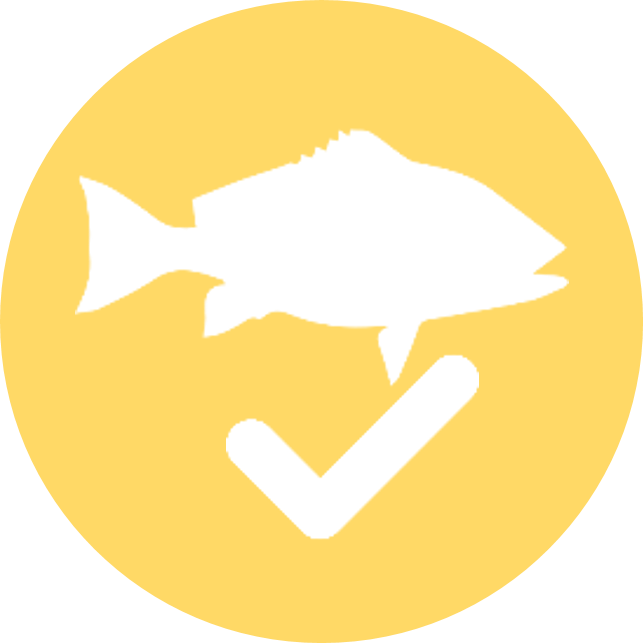
Format & visualise fish data
Claude Spencer & Brooke Gibbons
2023-11-13
format-visualise-fish.Rmd
This script takes the checked fish data from the previous workflow steps, visualises the data and exports it into a format suitable for modelling. The exploratory visualisation of the data allows for trends and patterns in the raw data to be investigated.
R set up
Load the necessary libraries.
library('remotes')
options(timeout=9999999)
# remotes::install_github("GlobalArchiveManual/CheckEM")
library(CheckEM)
library(tidyverse)
library(ggplot2)
library(sf)
library(here)
library(leaflet)
Set the study name.
name <- "example-bruv-workflow"
Read in the data
Load and format the metadata.
metadata.bathy.derivatives <- readRDS(here::here(paste0("r-workflows/data/tidy/", name, "_metadata-bathymetry-derivatives.rds"))) %>%
glimpse()
## Rows: 32
## Columns: 22
## $ campaignid <chr> "2023-03_SwC_stereo-BRUVs", "2023-03_SwC_s…
## $ sample <chr> "35", "5", "26", "23", "29", "4", "32", "3…
## $ date_time <chr> "14/03/2023 23:36", "14/03/2023 23:49", "1…
## $ location <chr> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA…
## $ site <chr> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA…
## $ depth <chr> "39.6", "42.7", "36", "41", "42.6", "45", …
## $ successful_count <chr> "Yes", "Yes", "Yes", "Yes", "Yes", "Yes", …
## $ successful_length <chr> "Yes", "Yes", "Yes", "Yes", "Yes", "Yes", …
## $ successful_habitat_forward <chr> "Yes", "Yes", "Yes", "Yes", "Yes", "Yes", …
## $ successful_habitat_backward <chr> "Yes", "Yes", "Yes", "Yes", "Yes", "Yes", …
## $ x <dbl> 114.9236, 114.9292, 114.9284, 114.9190, 11…
## $ y <dbl> -34.13155, -34.12953, -34.12729, -34.12832…
## $ longitude_dd <dbl> 114.9236, 114.9292, 114.9284, 114.9190, 11…
## $ latitude_dd <dbl> -34.13155, -34.12953, -34.12729, -34.12832…
## $ ID <dbl> 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73…
## $ mbdepth <dbl> -34.97151, -36.35807, -40.68553, -38.25594…
## $ slope <dbl> 0.146843375, 0.812689749, 0.694289634, 0.4…
## $ aspect <dbl> 209.89577, 62.41434, 40.87387, 294.10675, …
## $ TPI <dbl> 0.42153454, 2.39535522, -0.67607403, 0.476…
## $ TRI <dbl> 0.75557327, 3.29823494, 2.39221191, 1.8367…
## $ roughness <dbl> 2.21119308, 8.36493301, 8.36493301, 5.3012…
## $ detrended <dbl> -5.6631737, -7.0394716, -11.2637815, -8.69…
Load and format the habitat data. Here we create a ‘reef’ column that consists of reef-forming habitat found in the study area, which will be used in subsequent fish modelling scripts.
habitat <- readRDS(here::here(paste0("r-workflows/data/tidy/", name, "_tidy-habitat.rds"))) %>%
dplyr::mutate(number = number / total_points_annotated) %>%
dplyr::select(campaignid, sample, longitude_dd, latitude_dd, depth, mbdepth, slope, aspect, tpi, tri, roughness, detrended, habitat, number, mean_relief, sd_relief) %>%
pivot_wider(names_from = habitat, values_from = number, values_fill = 0) %>%
CheckEM::clean_names() %>%
dplyr::mutate(reef = consolidated_hard + macroalgae + seagrasses + sessile_invertebrates) %>%
dplyr::select(-c(consolidated_hard, macroalgae, seagrasses, sessile_invertebrates, unconsolidated_soft)) %>%
glimpse()
## Rows: 32
## Columns: 15
## $ campaignid <chr> "2023-03_SwC_stereo-BRUVs", "2023-03_SwC_stereo-BRUVs", "…
## $ sample <chr> "35", "5", "26", "23", "29", "4", "32", "37", "3", "2", "…
## $ longitude_dd <dbl> 114.9236, 114.9292, 114.9284, 114.9190, 114.9105, 114.911…
## $ latitude_dd <dbl> -34.13155, -34.12953, -34.12729, -34.12832, -34.12296, -3…
## $ depth <chr> "39.6", "42.7", "36", "41", "42.6", "45", "44.4", "46.1",…
## $ mbdepth <dbl> -34.97151, -36.35807, -40.68553, -38.25594, -39.91339, -4…
## $ slope <dbl> 0.146843375, 0.812689749, 0.694289634, 0.444838455, 0.322…
## $ aspect <dbl> 209.89577, 62.41434, 40.87387, 294.10675, 307.15484, 319.…
## $ tpi <dbl> 0.42153454, 2.39535522, -0.67607403, 0.47694588, 0.875010…
## $ tri <dbl> 0.75557327, 3.29823494, 2.39221191, 1.83670998, 1.3206706…
## $ roughness <dbl> 2.21119308, 8.36493301, 8.36493301, 5.30128479, 3.3831062…
## $ detrended <dbl> -5.6631737, -7.0394716, -11.2637815, -8.6934719, -9.84557…
## $ mean_relief <dbl> 3.034483, 3.900000, 4.000000, 3.555556, 4.000000, 3.76190…
## $ sd_relief <dbl> 1.1174831, 0.4472136, 0.0000000, 0.8555853, 0.0000000, 0.…
## $ reef <dbl> 0.9298246, 1.0000000, 1.0000000, 1.0000000, 1.0000000, 1.…
Load length of maturity data. The maturity dataset is loaded when the package is installed.
maturity.mean <- maturity %>%
dplyr::group_by(family, genus, species, sex) %>%
dplyr::slice(which.min(l50_mm)) %>%
ungroup() %>%
dplyr::group_by(family, genus, species) %>%
dplyr::summarise(l50 = mean(l50_mm)) %>%
ungroup() %>%
glimpse()
## `summarise()` has grouped output by 'family', 'genus'. You can override using
## the `.groups` argument.
## Rows: 42
## Columns: 4
## $ family <chr> "Arripidae", "Berycidae", "Carangidae", "Carangidae", "Carchar…
## $ genus <chr> "Arripis", "Centroberyx", "Pseudocaranx", "Seriola", "Carcharh…
## $ species <chr> "truttaceus", "gerrardi", "spp", "hippos", "obscurus", "plumbe…
## $ l50 <dbl> 600.0, 266.0, 310.0, 831.0, 2540.0, 1360.0, 670.0, 400.0, 466.…
Load and format count data, and transform this into total abundance and species richness. These two response variables will be used in subsequent fish modelling scripts.
tidy.count <- readRDS(here::here(paste0("r-workflows/data/staging/", name, "_complete-count.rds"))) %>%
dplyr::filter(campaignid %in% "2023-03_SwC_stereo-BRUVs") %>% # Remove Pt Cloates campaign for now
dplyr::group_by(campaignid, sample, status, scientific) %>%
dplyr::summarise(count = sum(count)) %>%
pivot_wider(names_from = "scientific", values_from = count, values_fill = 0) %>%
dplyr::ungroup() %>%
dplyr::mutate(total_abundance = rowSums(.[, 4:(ncol(.))], na.rm = TRUE), species_richness = rowSums(.[, 4:(ncol(.))] > 0)) %>%
dplyr::select(campaignid, sample, status, total_abundance, species_richness) %>%
pivot_longer(cols = c("total_abundance", "species_richness"), names_to = "response", values_to = "number") %>%
dplyr::left_join(habitat) %>%
glimpse()
## `summarise()` has grouped output by 'campaignid', 'sample', 'status'. You can
## override using the `.groups` argument.
## Joining with `by = join_by(campaignid, sample)`
## Rows: 64
## Columns: 18
## $ campaignid <chr> "2023-03_SwC_stereo-BRUVs", "2023-03_SwC_stereo-BRUVs", "…
## $ sample <chr> "10", "10", "12", "12", "14", "14", "15", "15", "16", "16…
## $ status <chr> "No-take", "No-take", "No-take", "No-take", "No-take", "N…
## $ response <chr> "total_abundance", "species_richness", "total_abundance",…
## $ number <dbl> 111, 13, 94, 15, 191, 9, 146, 16, 99, 14, 312, 18, 38, 13…
## $ longitude_dd <dbl> 114.8533, 114.8533, 114.8816, 114.8816, 114.8686, 114.868…
## $ latitude_dd <dbl> -34.08387, -34.08387, -34.13249, -34.13249, -34.07530, -3…
## $ depth <chr> "44.3", "44.3", "42.6", "42.6", "42.7", "42.7", "45.3", "…
## $ mbdepth <dbl> -42.77519, -42.77519, -42.02835, -42.02835, -41.07679, -4…
## $ slope <dbl> 0.005708777, 0.005708777, 0.013768068, 0.013768068, 0.078…
## $ aspect <dbl> 72.31942, 72.31942, 110.35012, 110.35012, 215.54254, 215.…
## $ tpi <dbl> 0.05208921, 0.05208921, 0.03892708, 0.03892708, 0.2611088…
## $ tri <dbl> 0.05208921, 0.05208921, 0.06246567, 0.06246567, 0.3096442…
## $ roughness <dbl> 0.11757660, 0.11757660, 0.30690765, 0.30690765, 0.7959022…
## $ detrended <dbl> -2.8542883, -2.8542883, -5.8822656, -5.8822656, -6.646017…
## $ mean_relief <dbl> 3.217391, 3.217391, 2.809524, 2.809524, 2.440000, 2.44000…
## $ sd_relief <dbl> 0.7952428, 0.7952428, 0.4023739, 0.4023739, 0.7118052, 0.…
## $ reef <dbl> 0.9690722, 0.9690722, 1.0000000, 1.0000000, 0.9420290, 0.…
Load and format length data.
lengths <- readRDS(here::here(paste0("r-workflows/data/staging/", name, "_complete-length.rds"))) %>%
dplyr::filter(campaignid %in% "2023-03_SwC_stereo-BRUVs") %>% # Remove Pt Cloates campaign for now
# dplyr::mutate(depth = as.numeric(depth)) %>%
left_join(habitat) %>%
left_join(maturity.mean) %>%
dplyr::mutate(number = 1) %>%
glimpse()
## Joining with `by = join_by(campaignid, sample)`
## Joining with `by = join_by(family, genus, species)`
## Rows: 9,867
## Columns: 35
## $ campaignid <chr> "2023-03_SwC_stereo-BRUVs", "2023-03_SwC_s…
## $ sample <chr> "10", "10", "10", "10", "10", "10", "10", …
## $ family <chr> "Acanthuridae", "Acanthuridae", "Acanthuri…
## $ genus <chr> "Naso", "Naso", "Naso", "Albula", "Aplodac…
## $ species <chr> "brachycentron", "fageni", "hexacanthus", …
## $ length_mm <dbl> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA…
## $ number <dbl> 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, …
## $ range <dbl> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA…
## $ rms <dbl> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA…
## $ precision <dbl> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA…
## $ status <chr> "No-take", "No-take", "No-take", "No-take"…
## $ date_time <chr> "18/03/2023 2:44", "18/03/2023 2:44", "18/…
## $ location <chr> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA…
## $ site <chr> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA…
## $ depth_m <chr> "44.3", "44.3", "44.3", "44.3", "44.3", "4…
## $ successful_count <chr> "Yes", "Yes", "Yes", "Yes", "Yes", "Yes", …
## $ successful_length <chr> "Yes", "Yes", "Yes", "Yes", "Yes", "Yes", …
## $ successful_habitat_forward <chr> "Yes", "Yes", "Yes", "Yes", "Yes", "Yes", …
## $ successful_habitat_backward <chr> "Yes", "Yes", "Yes", "Yes", "Yes", "Yes", …
## $ marine_region <chr> "South-west", "South-west", "South-west", …
## $ scientific <chr> "Acanthuridae Naso brachycentron", "Acanth…
## $ longitude_dd <dbl> 114.8533, 114.8533, 114.8533, 114.8533, 11…
## $ latitude_dd <dbl> -34.08387, -34.08387, -34.08387, -34.08387…
## $ depth <chr> "44.3", "44.3", "44.3", "44.3", "44.3", "4…
## $ mbdepth <dbl> -42.77519, -42.77519, -42.77519, -42.77519…
## $ slope <dbl> 0.005708777, 0.005708777, 0.005708777, 0.0…
## $ aspect <dbl> 72.31942, 72.31942, 72.31942, 72.31942, 72…
## $ tpi <dbl> 0.05208921, 0.05208921, 0.05208921, 0.0520…
## $ tri <dbl> 0.05208921, 0.05208921, 0.05208921, 0.0520…
## $ roughness <dbl> 0.1175766, 0.1175766, 0.1175766, 0.1175766…
## $ detrended <dbl> -2.854288, -2.854288, -2.854288, -2.854288…
## $ mean_relief <dbl> 3.217391, 3.217391, 3.217391, 3.217391, 3.…
## $ sd_relief <dbl> 0.7952428, 0.7952428, 0.7952428, 0.7952428…
## $ reef <dbl> 0.9690722, 0.9690722, 0.9690722, 0.9690722…
## $ l50 <dbl> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA…
Format data for visualisation and modelling
Filter length data to only include ‘indicator species’. In our case study, these are three species that have been defined as bioregional-scale demersal fish indicators by the West Australian Department of Primary Industries and Regional Development. If there is no equivalent in your study area, we suggest using ecologically-relevant and highly-targeted species.
indicator.species <- lengths %>%
dplyr::mutate(scientific = paste(genus, species, sep = " ")) %>%
dplyr::filter(scientific %in% c("Choerodon rubescens", "Chrysophrys auratus", "Glaucosoma hebraicum")) %>%
glimpse()
## Rows: 144
## Columns: 35
## $ campaignid <chr> "2023-03_SwC_stereo-BRUVs", "2023-03_SwC_s…
## $ sample <chr> "10", "10", "10", "12", "12", "12", "14", …
## $ family <chr> "Glaucosomatidae", "Labridae", "Sparidae",…
## $ genus <chr> "Glaucosoma", "Choerodon", "Chrysophrys", …
## $ species <chr> "hebraicum", "rubescens", "auratus", "hebr…
## $ length_mm <dbl> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA…
## $ number <dbl> 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, …
## $ range <dbl> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA…
## $ rms <dbl> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA…
## $ precision <dbl> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA…
## $ status <chr> "No-take", "No-take", "No-take", "No-take"…
## $ date_time <chr> "18/03/2023 2:44", "18/03/2023 2:44", "18/…
## $ location <chr> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA…
## $ site <chr> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA…
## $ depth_m <chr> "44.3", "44.3", "44.3", "42.6", "42.6", "4…
## $ successful_count <chr> "Yes", "Yes", "Yes", "Yes", "Yes", "Yes", …
## $ successful_length <chr> "Yes", "Yes", "Yes", "Yes", "Yes", "Yes", …
## $ successful_habitat_forward <chr> "Yes", "Yes", "Yes", "Yes", "Yes", "Yes", …
## $ successful_habitat_backward <chr> "Yes", "Yes", "Yes", "Yes", "Yes", "Yes", …
## $ marine_region <chr> "South-west", "South-west", "South-west", …
## $ scientific <chr> "Glaucosoma hebraicum", "Choerodon rubesce…
## $ longitude_dd <dbl> 114.8533, 114.8533, 114.8533, 114.8816, 11…
## $ latitude_dd <dbl> -34.08387, -34.08387, -34.08387, -34.13249…
## $ depth <chr> "44.3", "44.3", "44.3", "42.6", "42.6", "4…
## $ mbdepth <dbl> -42.77519, -42.77519, -42.77519, -42.02835…
## $ slope <dbl> 0.005708777, 0.005708777, 0.005708777, 0.0…
## $ aspect <dbl> 72.31942, 72.31942, 72.31942, 110.35012, 1…
## $ tpi <dbl> 0.05208921, 0.05208921, 0.05208921, 0.0389…
## $ tri <dbl> 0.05208921, 0.05208921, 0.05208921, 0.0624…
## $ roughness <dbl> 0.11757660, 0.11757660, 0.11757660, 0.3069…
## $ detrended <dbl> -2.8542883, -2.8542883, -2.8542883, -5.882…
## $ mean_relief <dbl> 3.217391, 3.217391, 3.217391, 2.809524, 2.…
## $ sd_relief <dbl> 0.7952428, 0.7952428, 0.7952428, 0.4023739…
## $ reef <dbl> 0.9690722, 0.9690722, 0.9690722, 1.0000000…
## $ l50 <dbl> 310.5, 379.0, 352.0, 310.5, 379.0, 352.0, …
Create a unique list of the samples per campaign, to fill the zeroes back into processed length data.
## Rows: 32
## Columns: 3
## $ campaignid <chr> "2023-03_SwC_stereo-BRUVs", "2023-03_SwC_stereo-BRUVs", "20…
## $ sample <chr> "10", "12", "14", "15", "16", "17", "19", "2", "21", "22", …
## $ status <chr> "No-take", "No-take", "No-take", "No-take", "No-take", "No-…
Create a dataframe of the indicator species greater than length of maturity.
greater.mat <- indicator.species %>%
dplyr::filter(length_mm > l50) %>%
dplyr::group_by(campaignid, sample) %>%
dplyr::summarise(number = sum(number)) %>%
ungroup() %>%
right_join(metadata.length) %>%
dplyr::mutate(number = ifelse(is.na(number), 0, number)) %>%
dplyr::mutate(response = "greater than Lm") %>%
left_join(habitat) %>%
dplyr::glimpse()
## `summarise()` has grouped output by 'campaignid'. You can override using the
## `.groups` argument.
## Joining with `by = join_by(campaignid, sample)`
## Joining with `by = join_by(campaignid, sample)`
## Rows: 32
## Columns: 18
## $ campaignid <chr> "2023-03_SwC_stereo-BRUVs", "2023-03_SwC_stereo-BRUVs", "…
## $ sample <chr> "15", "17", "19", "23", "24", "26", "29", "3", "31", "32"…
## $ number <dbl> 1, 1, 8, 3, 2, 3, 4, 1, 4, 6, 1, 3, 1, 2, 4, 2, 6, 1, 13,…
## $ status <chr> "No-take", "No-take", "No-take", "No-take", "No-take", "N…
## $ response <chr> "greater than Lm", "greater than Lm", "greater than Lm", …
## $ longitude_dd <dbl> 114.8444, 114.8576, 114.7822, 114.9190, 114.8485, 114.928…
## $ latitude_dd <dbl> -34.08478, -34.09635, -34.12047, -34.12832, -34.11789, -3…
## $ depth <chr> "45.3", "43.3", "73.6", "41", "45.6", "36", "42.6", "46.7…
## $ mbdepth <dbl> -44.29804, -42.74676, -71.60112, -38.25594, -44.23959, -4…
## $ slope <dbl> 0.108975978, 0.008719478, 0.880997142, 0.444838455, 0.103…
## $ aspect <dbl> 264.03427, 30.76545, 262.35388, 294.10675, 257.20307, 40.…
## $ tpi <dbl> -0.19527912, 0.02505112, 1.04176044, 0.47694588, 0.042278…
## $ tri <dbl> 0.34487247, 0.03473282, 2.80258274, 1.83670998, 0.3347983…
## $ roughness <dbl> 0.91366196, 0.09923935, 9.01136017, 5.30128479, 1.2933082…
## $ detrended <dbl> -0.2281526, -3.1375041, 17.9911461, -8.6934719, 2.9724391…
## $ mean_relief <dbl> 2.518519, 3.333333, 2.583333, 3.555556, 3.360000, 4.00000…
## $ sd_relief <dbl> 0.8024180, 0.9128709, 0.5036102, 0.8555853, 0.4898979, 0.…
## $ reef <dbl> 0.8292683, 1.0000000, 0.9493671, 1.0000000, 1.0000000, 1.…
Create a dataframe of the indicator species smaller than length of maturity.
smaller.mat <- indicator.species %>%
dplyr::filter(length_mm < l50) %>%
dplyr::group_by(campaignid, sample) %>%
dplyr::summarise(number = sum(number)) %>%
ungroup() %>%
right_join(metadata.length) %>%
dplyr::mutate(number = ifelse(is.na(number), 0, number)) %>%
dplyr::mutate(response = "smaller than Lm") %>%
left_join(habitat) %>%
dplyr::glimpse()
## `summarise()` has grouped output by 'campaignid'. You can override using the
## `.groups` argument.
## Joining with `by = join_by(campaignid, sample)`
## Joining with `by = join_by(campaignid, sample)`
## Rows: 32
## Columns: 18
## $ campaignid <chr> "2023-03_SwC_stereo-BRUVs", "2023-03_SwC_stereo-BRUVs", "…
## $ sample <chr> "26", "4", "7", "10", "12", "14", "15", "16", "17", "19",…
## $ number <dbl> 1, 1, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, …
## $ status <chr> "No-take", "No-take", "No-take", "No-take", "No-take", "N…
## $ response <chr> "smaller than Lm", "smaller than Lm", "smaller than Lm", …
## $ longitude_dd <dbl> 114.9284, 114.9112, 114.7854, 114.8533, 114.8816, 114.868…
## $ latitude_dd <dbl> -34.12729, -34.11597, -34.09623, -34.08387, -34.13249, -3…
## $ depth <chr> "36", "45", "62.7", "44.3", "42.6", "42.7", "45.3", "45.4…
## $ mbdepth <dbl> -40.68553, -42.80315, -60.38860, -42.77519, -42.02835, -4…
## $ slope <dbl> 0.694289634, 0.045436668, 0.115437569, 0.005708777, 0.013…
## $ aspect <dbl> 40.87387, 319.32290, 319.71953, 72.31942, 110.35012, 215.…
## $ tpi <dbl> -0.67607403, 0.17132854, 0.07896280, 0.05208921, 0.038927…
## $ tri <dbl> 2.39221191, 0.20512867, 0.37800837, 0.05208921, 0.0624656…
## $ roughness <dbl> 8.36493301, 0.54244614, 1.60873032, 0.11757660, 0.3069076…
## $ detrended <dbl> -11.2637815, -12.7394981, 19.9898605, -2.8542883, -5.8822…
## $ mean_relief <dbl> 4.000000, 3.761905, 3.000000, 3.217391, 2.809524, 2.44000…
## $ sd_relief <dbl> 0.0000000, 0.4364358, 0.0000000, 0.7952428, 0.4023739, 0.…
## $ reef <dbl> 1.0000000, 1.0000000, 1.0000000, 0.9690722, 1.0000000, 0.…
Join the two datasets.
## Rows: 64
## Columns: 18
## $ campaignid <chr> "2023-03_SwC_stereo-BRUVs", "2023-03_SwC_stereo-BRUVs", "…
## $ sample <chr> "15", "17", "19", "23", "24", "26", "29", "3", "31", "32"…
## $ number <dbl> 1, 1, 8, 3, 2, 3, 4, 1, 4, 6, 1, 3, 1, 2, 4, 2, 6, 1, 13,…
## $ status <chr> "No-take", "No-take", "No-take", "No-take", "No-take", "N…
## $ response <chr> "greater than Lm", "greater than Lm", "greater than Lm", …
## $ longitude_dd <dbl> 114.8444, 114.8576, 114.7822, 114.9190, 114.8485, 114.928…
## $ latitude_dd <dbl> -34.08478, -34.09635, -34.12047, -34.12832, -34.11789, -3…
## $ depth <chr> "45.3", "43.3", "73.6", "41", "45.6", "36", "42.6", "46.7…
## $ mbdepth <dbl> -44.29804, -42.74676, -71.60112, -38.25594, -44.23959, -4…
## $ slope <dbl> 0.108975978, 0.008719478, 0.880997142, 0.444838455, 0.103…
## $ aspect <dbl> 264.03427, 30.76545, 262.35388, 294.10675, 257.20307, 40.…
## $ tpi <dbl> -0.19527912, 0.02505112, 1.04176044, 0.47694588, 0.042278…
## $ tri <dbl> 0.34487247, 0.03473282, 2.80258274, 1.83670998, 0.3347983…
## $ roughness <dbl> 0.91366196, 0.09923935, 9.01136017, 5.30128479, 1.2933082…
## $ detrended <dbl> -0.2281526, -3.1375041, 17.9911461, -8.6934719, 2.9724391…
## $ mean_relief <dbl> 2.518519, 3.333333, 2.583333, 3.555556, 3.360000, 4.00000…
## $ sd_relief <dbl> 0.8024180, 0.9128709, 0.5036102, 0.8555853, 0.4898979, 0.…
## $ reef <dbl> 0.8292683, 1.0000000, 0.9493671, 1.0000000, 1.0000000, 1.…
Visualise the fish count data
Filter the count (maxn) data to only include one response. In this example the count metrics included are total abundance and species richness.
response.name <- 'total_abundance'
overzero <- tidy.count %>%
filter(response %in% response.name & number > 0)
equalzero <- tidy.count %>%
filter(response %in% response.name & number == 0)
Visualise the count data as a bubble plot. White bubbles represent zeroes and the size of blue bubbles represents abundance.
bubble.plot <- leaflet(data = tidy.count) %>%
addTiles() %>%
addProviderTiles('Esri.WorldImagery', group = "World Imagery") %>%
addLayersControl(baseGroups = c("Open Street Map", "World Imagery"), options = layersControlOptions(collapsed = FALSE))
if (nrow(overzero)) {
bubble.plot <- bubble.plot %>%
addCircleMarkers(data = overzero, lat = ~ latitude_dd, lng = ~ longitude_dd, radius = ~ number / 10, fillOpacity = 0.5, stroke = FALSE, label = ~ as.character(sample))}
if (nrow(equalzero)) {
bubble.plot <- bubble.plot %>%
addCircleMarkers(data = equalzero, lat = ~ latitude_dd, lng = ~ longitude_dd, radius = 2, fillOpacity = 0.5, color = "white", stroke = FALSE, label = ~ as.character(sample))}
bubble.plot
Visualise the fish length data
Filter the length data to only include one response. In this example the length metrics included are indicator species greater than and smaller than the length of maturity.
response.name <- 'greater than Lm'
overzero <- tidy.length %>%
dplyr::filter(response %in% response.name & number > 0)
equalzero <- tidy.length %>%
dplyr::filter(response %in% response.name & number == 0)
Visualise the count data as a bubble plot. White bubbles represent zeroes and the size of blue bubbles represents abundance.
bubble.plot <- leaflet(data = tidy.length) %>%
addTiles() %>%
addProviderTiles('Esri.WorldImagery', group = "World Imagery") %>%
addLayersControl(baseGroups = c("Open Street Map", "World Imagery"), options = layersControlOptions(collapsed = FALSE))
if (nrow(overzero)) {
bubble.plot <- bubble.plot %>%
addCircleMarkers(data = overzero, lat = ~ latitude_dd, lng = ~ longitude_dd, radius = ~ number + 3, fillOpacity = 0.5, stroke = FALSE, label = ~ as.character(sample))}
if (nrow(equalzero)) {
bubble.plot <- bubble.plot %>%
addCircleMarkers(data = equalzero, lat = ~ latitude_dd, lng = ~ longitude_dd, radius = 2, fillOpacity = 0.5, color = "white", stroke = FALSE, label = ~ as.character(sample))}
bubble.plot